The ResultSetMetaData interface in JDBC can be used to gather meta data about the result set. The meta data can include information like number of columns in a result set, type of a column, name of a column, table name, etc.
Some of the methods available in ResultSetMetaData interface are given below:
Method | Description |
public int getColumnCount() throws SQLException | Returns the number of columns in the result set |
public String getColumnName(int index) throws SQLException | Returns the column name of the given index |
public String getColumnTypeName(int index) throws SQLException | Returns the column data type of the given index |
public String getTableName(int index )throws SQLException | Returns the table name for the given index |
To get the required meta data of a result set we will use the getMetaData() method as shown in the below example JDBC program:
import java.sql.*;
public class GetData {
static final String DB_URL = "jdbc:mysql://localhost/sampledb";
static final String USER = "root";
static final String PASS = "123456";
static final String QUERY = "SELECT id, name, mobile FROM students";
public static void main(String[] args) {
// Open a connection
try
{
Connection conn = DriverManager.getConnection(DB_URL, USER, PASS);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(QUERY);
ResultSetMetaData rsmd=rs.getMetaData();
System.out.println("Total columns: " + rsmd.getColumnCount());
System.out.println("Column Name of 1st column: " + rsmd.getColumnName(1));
System.out.println("Column Type Name of 1st column: " + rsmd.getColumnTypeName(1));
}
catch (SQLException e) {
e.printStackTrace();
}
}
}
Output for the above program is given below:
Total columns: 3
Column Name of 1st column: id
Column Type Name of 1st column: INT
Note: The above compiles successfully only when we add the JAR file to the CLASSPATH environment variable as shown below:
For more information on JDBC, refer the following links:
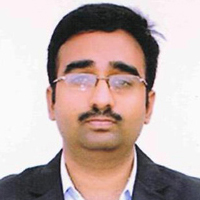
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply