This article explains about recursion in Java. We will learn what is recursion and how to use recursion to write effective Java programs.
Recursion is a famous way to solve problems with less lines of code. Many programmers prefer recursion over iteration as less amount of code is required to solve problems using recursion.
A method calling itself in its definition (body) is known as recursion. For implementing recursion, we need to follow the below requirements:
- There should be a base case where the recursion terminates and returns a value.
- The value of the parameter(s) should change in the recursive call. Otherwise, this leads to infinite loop.
Let’s consider the code for finding factorial of a number using iteration as shown below:
int fact(int n)
{
int r = 1;
for(int i = n; i > 1 ; i--)
{
r = i * r;
}
return r;
}
Now, let’s consider the recursive solution for finding the factorial of a given number which is shown below:
int rfact(int n)
{
if(n == 0 || n == 1)
return 1;
else
return n * rfact(n-1); //recursive call
}
The complete Java program which demonstrates recursion is given below:
class RecursionDemo
{
public static void main(String[] args)
{
int n = 6;
System.out.println("Factorial of " + n + " without recursion is: " + fact(n));
System.out.println("Factorial of " + n + " using recursion is: " + rfact(n));
}
static int fact(int n)
{
int r = 1;
for(int i = n; i > 1 ; i--)
{
r = i * r;
}
return r;
}
static int rfact(int n)
{
if(n == 0 || n == 1)
return 1;
else
return n * rfact(n-1); //recursive call
}
}
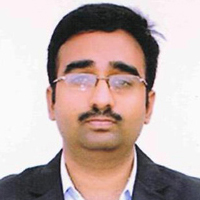
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply