In Java we can store objects as a byte stream and store it in files or send it over a network. The process of converting an object to a byte stream is serialization and the reverse process of converting a byte stream to an object is called deserialization.
In Java programs, we can achieve serialization and deserialization by using the object I/O classes: ObjectOutputStream and ObjectInputStream respectively. These classes are a part of java.io package. The base class for both these classes is InputStream.
ObjectOutputStream class
The ObjectOuputStream class serializes an object into byte stream to store in a file or to send it through a network. Some of the often-used methods in this class are described in the below table:
Method | Description |
void writeObject(Object) | Converts an object to byte stream |
void writeBoolean(boolean) | Converts a boolean to byte stream |
void writeByte(byte) | Converts a byte to byte stream |
void writeChar(char) | Converts a char to byte stream |
void writeDouble(double) | Converts a double to byte stream |
void writeFloat(float) | Converts a float to byte stream |
void writeInt(int) | Converts an int to byte stream |
void writeLong(long) | Converts a long to byte stream |
void writeShort(short) | Converts a short to byte stream |
Following Java program uses ObjectOutputStream class for writing an object to a file. We will also use Serializable interface which allows an object to be serialized.
import java.io.*;
class Student implements Serializable
{
int id;
String name;
Student(int id, String name)
{
this.id = id;
this.name = name;
}
public String toString()
{
return id + " - " + name;
}
}
public class Serialization
{
public static void main(String [] args) throws Exception
{
Student s = new Student(1, "Teja");
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("students.dat"));
oos.writeObject(s);
}
}
The output for the above program is, details of the student are stored in the file students.dat.
ObjectInputStream class
The ObjectInputStream class deserializes a byte stream from a file into object or primitive type data. Some of the often-used methods in this class are described in the below table:
Method | Description |
Object readObject() | Reads a byte stream and converts it to an object |
boolean writeBoolean() | Reads a byte stream and converts it to an boolean |
byte writeByte() | Reads a byte stream and converts it to an byte |
char writeChar() | Reads a byte stream and converts it to an char |
double writeDouble() | Reads a byte stream and converts it to an double |
float writeFloat() | Reads a byte stream and converts it to an float |
int writeInt() | Reads a byte stream and converts it to an int |
long writeLong() | Reads a byte stream and converts it to an long |
short writeShort() | Reads a byte stream and converts it to an short |
Following Java program uses ObjectInputStream class for reading a byte stream from a file and converting it into an object.
import java.io.*;
public class DeSerialization
{
public static void main(String [] args) throws Exception
{
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("students.dat"));
Student s = (Student) ois.readObject();
System.out.println(s);
}
}
Output for the above program is:
1 – Teja
For more information, visit the following links:
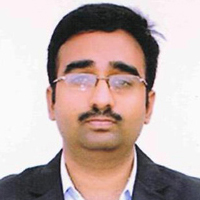
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply