In this article we will learn what are daemon threads? and how to make thread as daemon thread along with example Java program.
A daemon thread is a thread which runs in the background. Example for daemon thread in Java is the garbage collector. In Java, thread can be divided into two categories:
- User threads
- Daemon threads
A user thread is a general thread which is created by the user. A daemon thread is also a user thread which is made as a daemon thread (background thread).
Difference between a user thread and a daemon thread is, a Java program will not terminate if there is at least one user thread in execution. But, a Java program can terminate if there are one or more daemon threads in execution.
To work with daemon threads, Java provides two methods:
void setDaemon(boolean flag)
boolean isDaemon()
The setDaemon() method is used to convert a user thread into a daemon thread. The isDaemon() thread can be used to know whether a thread is a daemon thread or not.
Below program demonstrates a daemon thread:
class ChildThread implements Runnable
{
Thread t;
ChildThread(String name)
{
t = new Thread(this, name);
t.setDaemon(true);
t.start();
}
public void run()
{
try
{
while(true)
{
System.out.println("This thread is a daemon thread: "+t.isDaemon());
System.out.println(t.getName()+": Hi");
Thread.sleep(1000);
}
}
catch(InterruptedException e)
{
System.out.println("Child thread is interrupted");
}
}
}
class DaemonThread
{
public static void main(String args[])
{
ChildThread one = new ChildThread("Daemon Thread");
}
}
Output of the above program is:
This thread is a daemon thread: true
Daemon Thread: Hi
Daemon Thread: Hi
Daemon Thread: Hi
Daemon Thread: Hi
…
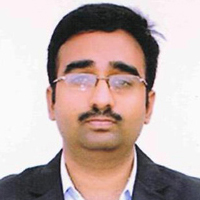
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply