Aim
To write a program for demonstrating the basic structure of a Java program.
Theory
A Java program is a collection of one or more classes, in which, one class contains the main method. Before looking at the structure of a Java program, let’s see the structure of a Java class. Structure of a Java class is as shown below:
Package Statement [Optional] [Must be the first line if written. Only exception is comment.]
Import Statement [Optional]
Comments [Optional] [Can be written anywhere in the code]
Class Declaration
{
Variable declarations
Comments
Constructors
Methods
Nested Classes
Nested Interfaces
Enums
}
A Java class can contain the following:
Package statement: A package statement is used to declare a Java class as a part of the specified package. Package statement is optional. When we decide to write a package statement, it should be the first statement in the file. Only exception to this rule is writing comments.
Import statement(s): We can write one or more import statements. These are also optional. An import statement is used to link our class with other classes in the same package or other packages to use their functionality.
Comments: We can write one or more comments in a Java class to explain the use of certain statements or provide extra information like purpose of the class, author’s name, date and time of creation etc. Comments are optional. Comments can be written in the first line or anywhere in the program.
Class declaration: A class declaration consists of the class keyword followed by the class name, which is followed by the body of the class represented using braces { }. A class declaration can contain the following:
- Variable declarations
- Comments
- Constructors
- Methods
- Nested classes
- Nested interfaces
- Enumerations
All the above elements (variable declarations, comments etc.) can occur in any order.
Now, a Java program contains one or more classes in which one class should be a driver class. A driver class is one which contains the main( ) function.
Pseudo code/Steps
- Create a class Student with member variables name, age, branch, grade.
- Create member methods as required.
- Save the file as java.
- Create a class Driver and create the object for Student
- Create a main
- Read the data and print the data by calling the methods on Student class object.
- Save the file as Driver.java
- Compile the program by using the command javac Driver.java.
- Run the program by using the command java Driver.
Program
Following is a Java program that demonstrates the structure of a Java program:
Student.java
class Student
{
String name;
int age;
String branch;
char grade;
Student(){}
Student(String n, int a, String b, char g)
{
name = n;
age = a;
branch = b;
grade = g;
}
void setName(String n)
{
name = n;
}
void setAge(int a)
{
age = a;
}
void setBranch(String b)
{
branch = b;
}
void setGrade(char g)
{
grade = g;
}
void getDetails()
{
System.out.println("Student name is: " + name);
System.out.println("Student age is: " + age);
System.out.println("Student branch is: " + branch);
System.out.println("Student grade is: " + grade);
}
}
Driver.java
import java.util.Scanner;
class Driver
{
public static void main(String[] args)
{
Scanner s = new Scanner(System.in);
System.out.println("Enter student details: ");
System.out.println("Enter student name: ");
String name = s.next();
System.out.println("Enter student age: ");
int age = s.nextInt();
System.out.println("Enter student branch: ");
String branch = s.next();
System.out.println("Enter student grade: ");
char grade = s.next().charAt(0);
Student s1 = new Student(name, age, branch, grade);
s1.getDetails();
}
}
Input and Output:
Enter student details:
Enter student name:
Suresh
Enter student age:
20
Enter student branch:
CSSE
Enter student grade:
A
Student name is: Suresh
Student age is: 20
Student branch is: CSSE
Student grade is: A
Result
Structure of a Java program was successfully demonstrated.
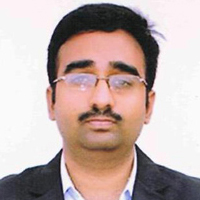
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply