This article provides a comprehensive overview of operators in Python programming language along with relevant examples.
Following are different types of operators in Python:
- Arithmetic operators
- Relational operators
- Assignment operators
- Logical operators
- Bitwise operators
- Membership operators
- Identity operators
Contents
Arithmetic Operators
Following are various arithmetic operators available in Python:
Operator | Description | Example |
+ | Add two operands | x+y = 26 |
– | Subtract one operand from another | x-y = 14 |
* | Multiply one operand with another | x*y = 120 |
/ | Divide one operand with another | x/y = 3.333 |
% | Remainder of division between two operands | x%y = 2 |
** | x to the power of y | x**y = 64000000 |
// | Floor division. Removes decimal part after division. | x//y = 3 |
Note: In above examples, x = 20 and y = 6
Relational Operators
Following are various relational operators available in Python:
Operator | Description | Example |
= = | Returns true if values of both operands are equal . Otherwise false. | x==y is false |
!= | Returns true if values of both operands are not equal. Otherwise false. | x!=y is true |
< > | Returns true if values of both operands are not equal. Otherwise false. | x!=y is true |
> | Returns true if left operand is greater than the right operand. Otherwise false. | x>y is true |
< | Returns true if left operand is less than the right operand. Otherwise false. | x<y is false |
>= | Returns true if left operand is greater than or equal to the right operand. Otherwise false. | x>=y is true |
<= | Returns true if left operand is less than or equal to the right operand. Otherwise false. | x<=y is false |
Note: In above examples, x = 20 and y = 6
Assignment Operators
Following are various assignment operators available in Python:
Operator | Description | Example |
= | Assigns value of right side operand to left side operand | z=x; z=20 |
+= | Adds operand on left side with operand on right side and assigns the value to left side operand. | z+=x; z=20 |
-= | Subtracts operand on right side with operand on left side and assigns the value to left side operand. | z-=x; z=-20 |
*= | Multiplies operand on left side with operand on right side and assigns the value to left side operand. | z*=x; z=0 |
/= | Divide operand on left side with operand on right side and assigns the value to left side operand. | z/=x; z=0.0 |
%= | Assigns remainder of division to left side operand. | z%=x; z=0 |
**= | Assigns left operand power right operand to left side operand. | z**=x; z=0 |
//= | Assigns result of floor division to left side operand. | z//=x; z=0 |
Note: In above examples, x = 20 , y = 6, and z = 0
Bitwise Operators
Following are various bitwise operators available in Python:
Operator | Description | Example |
& (and) | Performs bit-wise and of both operands | x&y = 1 |
| (or) | Performs bit-wise or of both operands | x|y = 5 |
^ (ex-or) | Performs exclusive-or of both operands | x^y = 4 |
~ | Performs 1’s complement of the operand | ~x = -6 |
<< | Performs left shift of the left operand by n number of times | x<<2 = 20 |
>> | Performs right shift of the left operand by n number of times | x>>1 = 2 |
Note: In above examples, x = 5 , y = 1
Logical Operators
Following are various logical operators available in Python:
Operator | Description | Example |
and | If both left side and right side expressions are true, it returns true. Otherwise, false. | True and True is True |
or | If either or both of left side and right side expressions are true, it returns true. Otherwise, false. | True or False is True |
not | If expression evaluates to true, it returns false or if the expression evaluates to false, it returns true. | not True is False |
Membership Operators
Following are the membership operators available in Python:
Operator | Description | Example |
in | Evaluates to true if it finds a variable or value in the given sequence. Otherwise, it returns true. | y in x is True |
not in | Evaluates to true if it doesn’t find a variable or value in the given sequence. Otherwise, it returns false. | y not in x is False |
Note: In above examples, x = [1,2,3,4,5,6] , y = 2
Identity Operators
Following are the identity operators available in Python:
Operator | Description | Example |
is | Evaluates to true if both the variables point to the same object in memory. | x is y returns True |
is not | Evaluates to true if both the variables does not point to the same object in memory | x is not y returns False |
Note: In above examples, x = 10, y = x
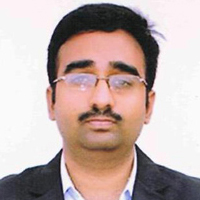
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply