In this article we will learn to implement a Java program for sorting a given list of names in ascending order. A Java program is provided for sorting a given list of names or strings in ascending order.
Program is as follows:
import java.util.*;
class Sorting
{
void sortStrings()
{
Scanner s = new Scanner(System.in);
System.out.println("Enter the value of n: ");
int n = s.nextInt();
String[] str = new String[n];
System.out.println("Enter strings: ");
for(int i = 0; i < n; i++)
{
str[i] = new String(s.next());
}
for(int i = 0; i < n; i++)
{
for(int j = i+1; j < n; j++)
{
if(str[i].compareTo(str[j])>0)
{
String temp = str[i];
str[i] = str[j];
str[j] = temp;
}
}
}
System.out.println("Sorted list of strings is:");
for(int i = 0; i < n ; i++)
{
System.out.println(str[i]);
}
}
}
class Driver
{
public static void main(String[] args)
{
Sorting obj = new Sorting();
obj.sortStrings();
}
}
Input and output for the above program are as follows:
Enter the value of n:
4
Enter strings:
suresh
mahesh
dinesh
ganesh
Sorted list of strings is:
dinesh
ganesh
mahesh
suresh
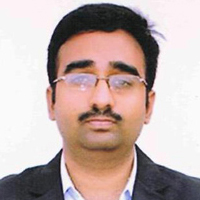
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply