In this article we will learn to implement a Java program to demonstrate life cycle of a thread. A Java program is provided below to illustrate life cycle of a thread.
Program is as follows:
import java.util.*;
class ChildThread implements Runnable
{
Thread t;
ChildThread()
{
t = new Thread(this);
System.out.println("Thread is in new state");
t.start();
}
public void run()
{
System.out.println("Thread is in runnable state");
Scanner s = new Scanner(System.in);
System.out.println("Thread entering blocked state");
System.out.print("Enter a string: ");
String str = s.next();
for(int i = 1; i <= 3; i++)
{
try
{
System.out.println("Thread suspended");
t.sleep(1000);
System.out.println("Thread is running");
}
catch(InterruptedException e)
{
System.out.println("Thread interrupted");
}
System.out.println("Thread terminated");
t.stop();
}
}
}
class Driver
{
public static void main(String[] args)
{
new ChildThread();
}
}
Output of the above program is as follows:
Thread is in new state
Thread is in runnable state
Thread entering blocked state
Enter a string: hai
Thread suspended
Thread is running
Thread terminated
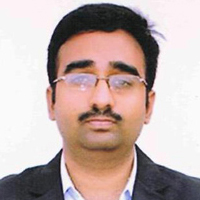
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply