Contents
Aim
To write a program for demonstrating packages using Java.
Theory
A package is a group of related classes. A package is used to restrict access to a class and to create namespaces. If all reasonable class names are already used, then we can create a new package (new namespace) and reuse the class names again.
For example, a package mypackage1 can contain a class named MyClass and another package mypackage2 can also contain a class named MyClass.
Defining or Creating a Package
A package can be created by including the package statement as first line in a Java source file. Syntax for creating or defining a package is given below:
package mypackage;
Multiple source files can include the package statement with the same package name. Packages are maintained as regular directories (folders) in the machine. For example, we have to create a folder named mypackage and store the .class files in that folder.
We can also create a hierarchy of packages as shown below:
package pack1.pack2.pack3;
Remember that packages are normal folders in the file system. So, pack3 is a sub folder in pack2 and pack2 is a sub folder in pack1.
Java Packages and CLASSPATH
It is not mandatory that the driver program (main program) and the package(s) to be at the same location. So how does JVM know the path to the package(s)?
There are three options:
- Placing the package in the current working directory.
- Specifying the package(s) path using CLASSPATH environment variable.
- Using the -classpath or -cp options with javac and java commands.
Importing or Using Packages
There are multiple ways in which we can import or use a package. They are as follows:
- Importing all the classes in a package.
- Importing only necessary classes in a package.
- Specifying the fully qualified name of the class.
Example for Creating and Using Packages
Consider the following hierarchy of folders and files.
The code for A.java, B.java, and C.java files is as given below:
A.java
package mypackage;
public class A
{
public void m1()
{
System.out.println("Class A method");
}
}
B.java
package mypackage;
public class B
{
public void m1()
{
System.out.println("Class B method");
}
}
C.java
package mypackage;
public class C
{
public void m1()
{
System.out.println("Class C method");
}
}
Now, we will compile A.java, B.java, and C.java files and place the generated A.class, B.class, and C.class files in the mypackage folder as shown in the figure above.
The code for PackageDemo.java file is as given below:
PackageDemo.java
import mypackage.A;
import mypackage.B;
import mypackage.C;
class Driver
{
public static void main(String args[])
{
A obj1 = new A();
obj1.m1();
B obj2 = new B();
obj2.m1();
C obj3 = new C();
obj3.m1();
}
}
Now, we can compile and run the above program with the following commands:
javac PackageDemo.java
java Driver
Let us change the location of mypackage directory now. So, consider the following hierarchy of folders and files.
Now we can use the following commands to compile and run the program:
set CLASSPATH=.;D:\
javac PackageDemo.java
java Driver
or we can also use the following commands to compile and run the program.
javac -cp .;D:\ PackageDemo.java
java -cp .;D:\ Driver
Pseudo code / Steps
- Create a package named myshapes.
- Create java, and Circle.java in the myshapes package.
- Create java file, where we will create the main() method.
- In the main() method create objects for the Rectangle, and Circle classes and call the area() and perimeter()
Program
Student has to write the program.
Input and Output:
Student has to write the input and output of the program.
Result
The concept of the packages has been understood and implemented successfully.
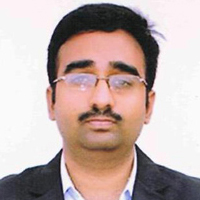
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply