Aim
To write a program for demonstrating methods and method overloading.
Theory
One of the ways through which Java supports polymorphism is overloading. It can be defined as creating two or more methods in the same class sharing a common name but different number of parameters or different types of parameters.
You should remember that overloading doesn’t depend upon the return type of the method. Since method binding is resolved at compile-time based on the number of parameters or type of parameters, overloading is also called as compile-time polymorphism or static binding or early binding.
Creating two or more methods in the same class with same name but different number of parameters or different types of parameters is known as method overloading. Following code segment demonstrates method overloading.
class Addition
{
void sum(int a, int b)
{
System.out.println("Sum of two integers is: "+(a+b));
}
void sum(float a, float b)
{
System.out.println("Sum of two floats is: "+(a+b));
}
}
Pseudo code / Steps
- Create a class
- Create the data members, namely, name, age, and grade.
- Create a method named setDetails for setting or assign the values for the data members.
- Overload the setDetails
- Create a method named getDetails for displaying the values of data members.
- Create a main method for creating objects and calling the overloaded method.
Program
class Student
{
String name;
int age;
char grade;
void setDetails(String n)
{
name = n;
}
void setDetails(String n, int a)
{
name = n;
age = a;
}
void setDetails(String n, int a, char g)
{
name = n;
age = a;
grade = g;
}
void getDetails()
{
System.out.println("Student name is " + name);
System.out.println("Student age is " + age);
System.out.println("Student grade is " + grade);
}
public static void main(String args[])
{
Student s1 = new Student();
s1.setDetails("Ram");
s1.getDetails();
s1.setDetails("Ram", 19);
s1.getDetails();
s1.setDetails("Ram", 19, 'A');
s1.getDetails();
}
}
Input and Output:
Student name is Ram
Student age is 0
Student grade is
Student name is Ram
Student age is 19
Student grade is
Student name is Ram
Student age is 19
Student grade is A
Result
The concept of method overloading was understood and demonstrated successfully.
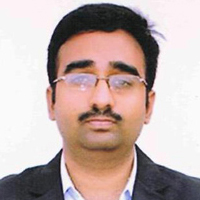
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply