Contents
Aim
To write a program for demonstrating layout managers using Java.
Theory
Java provides javax.swing package which does not need any help of the native operating system for creating GUI controls. Hence programs created using swings are portable.
Swings offers the following features:
- Lightweight Components: The components created using swings package doesn’t need the help of native operating system. Swings is a part of Java Foundation Classes (JFC) which are purely developed in Java. Also swing components require less memory and CPU cycles than their counterpart AWT components. Hence swing components are called lightweight components.
- Pluggable Look and Feel: Swings supports pluggable look and feel i.e., the appearance of a component can be separated from how the component behaves. This enables programmers to assign different look (themes) for the same component without changing its behavior.
Components and Containers
A swing GUI contains two main elements: components and containers. A component is an individual control like a button or label. A container is an object which can hold other components and containers.
Components
Swing components are derived from the class JComponent (except the four top-level containers). JComponent supports pluggable look and feel and supports the functionality common for all the components. JComponent class inherits the AWT classes Container and Component.
All swing components are represented as classes and are present in javax.swing package.
Containers
Swing provides two types of containers. First are top-level containers also called heavyweight containers. These are at the top in the containment hierarchy. The top-level containers are JFrame, JApplet, JWindow, and JDialog. All the containers inherit the AWT classes Component and Container.
Second are the lightweight containers which can be nested inside top-level containers. Lightweight containers are inherited from the class JComponent. Example of a lightweight container is JPanel.
Layout Managers
A layout manager is one which automatically manages the arrangement of various of components in a container. Each container will have a default layout manager.
The layout manager can be set by using the setLayout() method whose general form is as follows:
void setLayout(LayoutManager layoutObj)
We can manually arrange the position of each component (not recommended) by passing null to setLayout() method and by using setBounds() method on each component. Different layout managers available are:
- FlowLayout
- BorderLayout
- GridLayout
- CardLayout
- GridBagLayout
FlowLayout Manager
The flow layout manager arranges the components one after another from left-to-right and top-to-bottom manner. The flow layout manager gives some space between components. Flow layout manager instance can be created using anyone of the following constructors:
- FlowLayout()
- FlowLayout(int how)
- FlowLayout(int how, int hspace, int vspace)
In the above constructors, how specifies the alignment, hspace specifies horizontal space, and vspace specifies vertical space.
Pseudo code / Steps
- Create a class which extends the JFrame class available swing package.
- Create a button and label. Add them to the frame by setting the layout using FlowLayout
- The label should not be displayed by default.
- When the user clicks the button, the label should display some text message. Use ActionListener interface and override the action-Performed() method for this purpose.
Program
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class MyFrame extends JFrame implements ActionListener
{
JButton jb;
JLabel jl;
MyFrame()
{
setSize(500, 300);
setTitle("Flow Layout Demo");
setLayout(new FlowLayout());
jb = new JButton("Click Here");
jl = new JLabel();
add(jb);
add(jl);
jb.addActionListener(this);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void actionPerformed(ActionEvent e)
{
jl.setText("This is a label");
}
public static void main(String args[])
{
new MyFrame();
}
}
Input and Output:
Before button click
After button click
Result
The concepts related to layout managers were understood and implemented successfully.
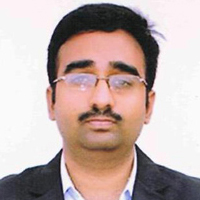
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply