Contents
Aim
To write a program for demonstrating inheritance and method overriding.
Theory
Inheritance
Inheritance is defined as: Deriving properties and behavior of one class to another class. The class from which things are derived is known as super class and the class in to which things are derived to is known as sub class. A super class can also be called as base or parent class and a sub class can also be called as derived or child class.
Inheritance relationship can be represented as shown below. In the figure, class A is super class and class B is sub class. In Java programs, inheritance is implemented using extends keyword. For example, if a class B wants to derive the characteristics of class A, we can write as shown below:
class B extends class A
{
//Members of class B
}
Method Overriding
Method overriding is possible only when the following things are satisfied:
- A class inherits from another class (inheritance).
- Both super class and sub class should contain a method with same signature.
Note: Method overriding does not depend up on the return type of the method and the access specifiers like (public, private, protected etc..).
When both super class and sub class contains the same method with same signature, and when we create an object for the sub class and call the method, the method in super class is overridden (or hidden) and the method in sub class gets called. This process is known as method overriding.
Dynamic Method Dispatch
Dynamic method dispatch is a mechanism which resolves the call to an overridden method at run-time based on the type of object being referred.
It is possible only when the following are satisfied:
- A class inherits from another class (inheritance)
- Super class variable refers a sub class object
- An overridden method is invoked using the super class reference
Pseudo code / Steps
- Create a class Shape which contains area and perimeter
- Create a sub class named Rectangle for the class Shape. Create two data members named length and breadth in Rectangle Create a constructor for Rectangle class. Override the area and perimeter methods of the super class.
- Create a sub class named Circle for the class Shape. Create a data member named radius in Circle Create a constructor for Circle class. Override the area and perimeter methods of the super class.
- Create a Driver class which contains the main The code for Driver class is provided below.
Program
class Shape
{
void area()
{
System.out.println("Area of shape");
}
void perimeter()
{
System.out.println("Perimeter of shape");
}
}
class Rectangle extends Shape
{
int length, breadth;
Rectangle(int l, int b)
{
length = l;
breadth = b;
}
void area()
{
System.out.println("Area of rectangle = " + (length*breadth));
}
void perimeter()
{
System.out.println("Perimeter of rectangle = " + 2*(length+breadth));
}
}
class Circle extends Shape
{
float radius;
Circle(float r)
{
radius = r;
}
void area()
{
System.out.println("Area of circle = " + (Math.PI*radius*radius));
}
void perimeter()
{
System.out.println("Perimeter of circle = " + (2*Math.PI*radius));
}
}
class Driver
{
public static void main(String args[])
{
Shape s = new Shape();
s.area();
s.perimeter();
Rectangle r = new Rectangle(20, 10);
r.area();
r.perimeter();
Circle c = new Circle(10.5f);
c.area();
c.perimeter();
Shape s1 = new Rectangle(30, 20);
s1.area();
s1.perimeter();
s = new Circle(2.5f);
s1.area();
s1.perimeter();
}
}
Input and Output:
Area of shape
Perimeter of shape
Area of rectangle = 200
Perimeter of rectangle = 60
Area of circle = 346.36059005827474
Perimeter of circle = 65.97344572538566
Area of rectangle = 600
Perimeter of rectangle = 100
Area of circle = 19.634954084936208
Perimeter of circle = 15.707963267948966
Result
The concepts of inheritance and method overriding were understood and successfully implemented.
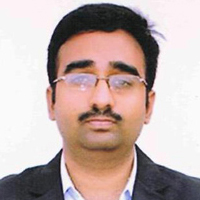
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply