In this article we will learn to implement a Java program to find the maximum and minimum value of an array. A java program is provided below which reads array elements from the user and finds out the maximum, minimum elements in the array.
Program is as follows:
import java.util.Scanner;
public class Driver
{
public static void main(String[] args)
{
Scanner input = new Scanner(System.in);
System.out.println("Enter the no. of elements: ");
int n = input.nextInt();
int a[] = new int[n];
System.out.println("Enter " + n + " numbers:");
for(int i = 0; i < n; i++)
a[i] = input.nextInt();
int max = a[0], min = a[0];
for(int i = 1; i < n; i++)
{
if(max < a[i])
max = a[i];
if(min > a[i])
min = a[i];
}
System.out.println("Max. number in the array is: " + max);
System.out.println("Min. number in the array is: " + min);
input.close();
}
}
Input and output for the above program are as follows:
Enter the no. of elements:
5
Enter 5 numbers:
1 6 8 -1 -2
Max. number in the array is: 8
Min. number in the array is: -2
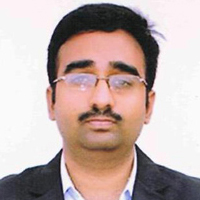
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply