In this article we will learn to implement a Java program for displaying file information. A Java program is provided below which takes file name as input and displays its contents.
Java program that reads a file name from the user and then displays information about whether the file exists, whether the file is readable/writable, the type of file and the length of the file in bytes and display the content using FileInputStream.
Program is as follows:
import java.io.*;
import javax.swing.*;
class FileDemo
{
public static void main(String args[])
{
String filename = JOptionPane.showInputDialog("Enter filename: ");
File f = new File(filename);
System.out.println("File exists: "+f.exists());
System.out.println("File is readable: "+f.canRead());
System.out.println("File is writable: "+f.canWrite());
System.out.println("Is a directory: "+f.isDirectory());
System.out.println("length of the file: "+f.length()+" bytes");
try
{
char ch;
StringBuffer buff = new StringBuffer("");
FileInputStream fis = new FileInputStream(filename);
while(fis.available()!=0)
{
ch = (char)fis.read();
buff.append(ch);
}
System.out.println("\nContents of the file are: ");
System.out.println(buff);
fis.close();
}
catch(FileNotFoundException e)
{
System.out.println("Cannot find the specified file...");
}
catch(IOException i)
{
System.out.println("Cannot read file...");
}
}
}
Input and output for the above program is as follows:
File name: sample.txt
File exists: true
File is readable: true
File is writable: true
Is a directory: false
length of the file: 20 bytes
Contents of the file are:
Hi, welcome to Java.
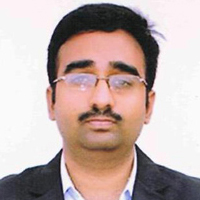
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply