In this article I will show you how to validate a HTML form using PHP and JavaScript. JavaScript performs preliminary checks on the client-side and PHP is used to validate the details entered by the user against the details available in the database on server-side.
AJAX has also been used to display the error message within the same webpage (form.html) without any redirections.
First let’s see the code of HTML form:
form.html
<!DOCTYPE html> <html> <head> <title>Login Form</title> </head> <body> <h2>Login Form</h2> <form action="validate.php" onsubmit="return validate()"> <p> <label>Username: </label> <input type="text" id="txtuser" name="txtuser" /> </p> <p> <label>Password: </label> <input type="password" id="txtpass" name="txtpass" /> </p> <p> <input type="submit" value="Login" /> <input type="reset" value="Clear" /> </p> </form> <p id="msg"></p> <script type="text/javascript" src="script2.js"></script> </body> </html>
JavaScript code to perform preliminary validations is as shown below:
script2.js
function validate() { var uname = document.getElementById("txtuser").value; var upass = document.getElementById("txtpass").value; xmlHttp = new XMLHttpRequest(); xmlHttp.onreadystatechange = processResponse; var url = "validate.php?uname="+uname+"&upass="+upass; xmlHttp.open("GET", url, true); xmlHttp.send(null); return false; } function processResponse() { if(xmlHttp.readyState==4 && xmlHttp.status==200) { var para = document.getElementById("msg"); para.innerHTML = xmlHttp.responseText; } }
Finally the PHP code to validate the user details against the data available in the database:
validate.php
<?php //$uname = $_GET["txtuser"]; //$upass = $_GET["txtpass"]; $uname = $_GET["uname"]; $upass = $_GET["upass"]; $flag = false; $con=mysqli_connect("localhost","root","123456","test"); $result = mysqli_query($con, "select * from users"); while($x = mysqli_fetch_array($result)) { if($uname == $x["uname"] && $upass == $x["upass"]) $flag = true; } if($flag) echo "Valid user!"; else echo "Invalid username or password!"; ?>
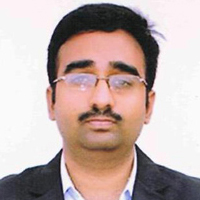
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply