Contents
Aim
To implement TCP congestion control algorithm.
S/W required
NS2, Xgraph
Background information
Congestion is an important factor in packet switched network. It refers to the state of a network where the message traffic becomes so heavy that the network response time slows down leading to the failure of the packets. It leads to packet loss. Due to this, it is necessary to control the congestion in the network, however, it cannot be avoided.
TCP congestion control refers to the mechanism that prevents congestion from happening or removes it after congestion takes place. When congestion takes place in the network, TCP handles it by reducing the size of the sender’s window. The window size of the sender is determined by the following two factors:
- Receiver window size
- Congestion window size
Receiver window size
It shows how much data can a receiver receive in bytes without giving any acknowledgment.
Things to remember for receiver window size:
- The sender should not send data greater than that of the size of receiver window.
- If the data sent is greater than that of the size of the receiver’s window, then it causes retransmission of TCP due to the dropping of TCP segment.
- Hence sender should always send data that is less than or equal to the size of the receiver’s window.
- TCP header is used for sending the window size of the receiver to the sender.
Congestion window size
It is the state of TCP that limits the amount of data to be sent by the sender into the network even before receiving the acknowledgment.
Following are the things to remember for the congestion window:
- To calculate the size of the congestion window, different variants of TCP and methods are used.
- Only the sender knows the congestion window and its size and it is not sent over the link or network.
The formula for determining the sender’s window size is:
Sender window size = Minimum (Receiver window size, Congestion window size)
Following are the causes of congestion in a network:
- Excessive consumption of bandwidth
- Improper subnet management
- Multicasting
- Outdated hardware
- Border gateway protocol
TCP Congestion Control
Congestion in TCP is handled by using these three phases:
- Slow Start
- Congestion Avoidance
- Congestion Detection
Slow Start Phase
In the slow start phase, the sender sets congestion window size = maximum segment size (1 MSS) at the initial stage. The sender increases the size of the congestion window by 1 MSS after receiving the ACK (acknowledgment). The size of the congestion window increases exponentially in this phase. The formula for determining the size of the congestion window is Congestion window size = Congestion window size + Maximum segment size.
This is how you calculate the size of the congestion window and it goes on for n number of values. The general formula for determining the size of the congestion window is (2) round trip time
This phase continues until window size reaches its slow start threshold.
The formula for determining the threshold is given:
Threshold = Maximum number of TCP segments that the receiver window can accommodate / 2
= (Receiver window size / Maximum Segment Size) / 2
Congestion Avoidance Phase
In this phase, after the threshold is reached, the size of the congestion window is increased by the sender linearly in order to avoid congestion. Each time an acknowledgment is received, the sender increments the size of the congestion window by 1.
The formula for determining the size of the congestion window in this phase is Congestion window size = Congestion window size + 1
This phase continues until the size of the window becomes equal to that of the receiver window size.
Congestion Detection Phase
In this phase, the sender identifies the segment loss and gives acknowledgment depending on the type of loss detected. There are two cases:
- Detection on time out.
- Detection on receiving 3 duplicate acknowledgements
Activity
Program
#create simulator
set ns [new Simulator]
#initialization script
set namfile [open out.nam w]
$ns namtrace-all $namfile
set outfile [open congestion.xg w]
#ermination script
proc finish {} {
global ns namfile
$ns flush-trace
close $namfile
exec nam out.nam &
#exec xgraph congestion.xg -geometry 300x300 &
exit 0
}
#to create nodes
set n0 [$ns node]
set n1 [$ns node]
set n2 [$ns node]
set n3 [$ns node]
set n4 [$ns node]
set n5 [$ns node]
# to create the link between the nodes with bandwidth, delay and queue
$ns duplex-link $n0 $n2 2Mb 10ms DropTail
$ns duplex-link $n1 $n2 2Mb 10ms DropTail
$ns duplex-link $n2 $n3 0.3Mb 200ms DropTail
$ns duplex-link $n3 $n4 0.5Mb 40ms DropTail
$ns duplex-link $n3 $n5 0.5Mb 30ms DropTail
# Sending node is 0 with agent as Reno Agent
set tcp1 [new Agent/TCP/Reno]
$ns attach-agent $n0 $tcp1
# receiving (sink) node is n4
set sink1 [new Agent/TCPSink]
$ns attach-agent $n4 $sink1
# establish the traffic between the source and sink
$ns connect $tcp1 $sink1
# Setup a FTP traffic generator on "tcp1"
set ftp1 [new Application/FTP]
$ftp1 attach-agent $tcp1
$ftp1 set type_ FTP
# procedure to plot the congestion window
proc plotWindow {tcpSource outfile} {
global ns
set now [$ns now]
set cwnd [$tcpSource set cwnd_]
# the data is recorded in a file called congestion.xg (this can be plotted # using xgraph or gnuplot. this example uses xgraph to plot the cwnd_
puts $outfile "$now $cwnd"
$ns at [expr $now+0.1] "plotWindow $tcpSource $outfile"
}
#timing events
$ns at 0.0 "plotWindow $tcp1 $outfile"
# start/stop the traffic
$ns at 0.1 "$ftp1 start"
$ns at 40.0 "$ftp1 stop"
# Set simulation end time
$ns at 50.0 "finish"
# Run simulation
$ns run
Input and output
Note: For observing output, we need to install xgraph, a third-party software. See the provided instructions in Google Classroom on how to install and configure xgraph for observing the output.
Output can be observed by using the following command:
Result
Congestion control algorithm is simulated and output is observed successfully.
References
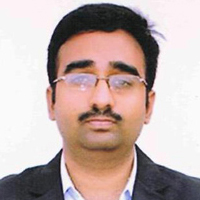
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply