Contents
Aim
To implement client-server communication using socket programming.
S/W required
Java
Background information
Client-Server communication model
One of the widely used way of communication across the Internet is the client-server communication. In this communication model, there is a system called server which always listens for connections from clients. Once a connection has been established, the server receives the requests from client and processes them.
On the other hand, a client is a system that can connect to a server when needed and sends requests to it. A request can be to access a file, data in a database, or some other resource that the server can provide.
Socket and port
A port is a point of communication. A PC or laptop has a total of 65,535 ports among which top 1024 ports are called as well-defined ports. These 1024 ports are used by different protocols for communication. For example, port 80 is the default port for HTTP. Every application which communicates with other applications across the Internet uses a port.
A socket is a combination of the IP address and a port. Generally, for developing a client-server application, we need to establish a connection through sockets for the purpose of communication.
Java socket programming
Java socket programming is used for allowing communication between applications running on JRE which are on different systems. Java’s socket programming can be connection-oriented or connectionless.
Socket and ServerSocket classes are used for connection-oriented socket programming and DatagramSocket and DatagramPacket classes are used for connectionless socket programming. Majority of the socket programming related classes are available in java.net package.
The server binds and listens to a port for connections from the clients. The accept() method of ServerSocket class blocks the console until the client is connected. After the successful connection of client, it returns the instance of Socket at server-side. The general process of client-server communication is as shown in the below figure:
Activity
Java Program
server.java
import java.net.*;
import java.io.*;
class NewServer
{
public static void main(String[] args) throws Exception
{
ServerSocket ss = new ServerSocket(6000);
Socket s = ss.accept();
DataInputStream dis = new DataInputStream(s.getInputStream());
DataOutputStream dos = new DataOutputStream(s.getOutputStream());
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String stream="";
String input="";
while(!stream.equals("stop"))
{
stream = dis.readUTF();
System.out.println("Client: " + stream);
input = br.readLine();
dos.writeUTF(input);
dos.flush();
}
dis.close();
dos.close();
s.close();
ss.close();
}
}
client.java
import java.net.*;
import java.io.*;
class NewClient
{
public static void main(String[] args) throws Exception
{
Socket s = new Socket("localhost", 6000);
DataInputStream dis = new DataInputStream(s.getInputStream());
DataOutputStream dos = new DataOutputStream(s.getOutputStream());
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
String stream="";
String input="";
while(!stream.equals("stop"))
{
input = br.readLine();
dos.writeUTF(input);
dos.flush();
stream = dis.readUTF();
System.out.println("Server: " + stream);
}
dis.close();
dos.close();
s.close();
}
}
Input and output
Result
Concepts of socket programming were understood and implemented successfully.
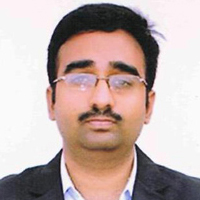
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply