In this article we will learn how to implement call by value and call by reference program in C++. A program in C++ is provided to implement call by value and call by reference using reference variable. Program is as follows:
#include <iostream>
using namespace std;
void swapval(int x, int y)
{
int temp;
temp = x;
x = y;
y = temp;
}
void swapref(int &x, int &y)
{
int temp;
temp = x;
x = y;
y = temp;
}
int main()
{
int a, b;
cout<<"Enter two numbers: ";
cin>>a>>b;
cout<<"Before swap a="<<a<<", b="<<b<<endl;
swapval(a,b);
cout<<"After swap by value a="<<a<<", b="<<b<<endl;
swapref(a,b);
cout<<"After swap by reference a="<<a<<", b="<<b<<endl;
return 0;
}
Input and output for the above program are as follows:
Enter two numbers: 10 20
Before swap a=10, b=20
After swap by value a=10, b=20
After swap by reference a=20, b=10
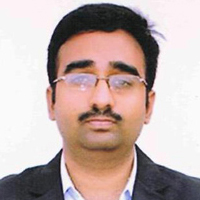
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply