In this article we will look at how to implement a CPP program to demonstrate runtime polymorphism. A C++ program is provided below to illustrate runtime polymorphism. Program is as follows:
#include <iostream>
using namespace std;
class Animal
{
public:
virtual void sound() = 0;
virtual void move() = 0;
};
class Dog : public Animal
{
public:
void sound()
{
cout<<"Bow wow wow"<<endl;
}
void move()
{
cout<<"Dog is moving"<<endl;
}
};
class Cat : public Animal
{
public:
void sound()
{
cout<<"Meow meow meow"<<endl;
}
void move()
{
cout<<"Cat is moving"<<endl;
}
};
int main()
{
Animal *a;
a = new Dog();
a->sound(); //run-time polymorphism
a = new Cat();
a->sound(); //run-time polymorphism
return 0;
}
Output for the above program is as follows:
Bow wow wow
Meow meow meow
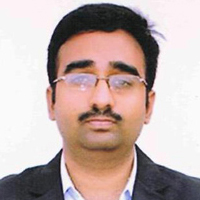
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply