In this article we will learn how to implement a pure virtual function program in C++. A C++ program is provided to illustrate the concept of pure virtual function and calculate the area of different shapes by using abstract class. Program is as follows:
#include <iostream>
using namespace std;
class Shape
{
public:
virtual void area() = 0;
};
class Rectangle : public Shape
{
private:
int l;
int b;
public:
Rectangle(int x, int y)
{
l = x;
b = y;
}
void area()
{
cout<<"Area of rectangle is: "<<(l*b)<<endl;
}
};
class Circle : public Shape
{
private:
int r;
public:
Circle(int x)
{
r = x;
}
void area()
{
cout<<"Area of circle is: "<<(3.142*r*r)<<endl;
}
};
int main()
{
Shape *s;
s = new Rectangle(10, 20);
s->area();
s = new Circle(2);
s->area();
return 0;
}
Output for the above program is as follows:
Area of rectangle is: 200
Area of circle is: 12.568
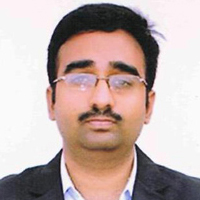
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply