In this article we will learn to implement a Cpp program demonstrating a bank account. A C++ program is provided below to represent a bank account.
Program is as follows:
#include <iostream>
using namespace std;
class Account
{
private:
string accno;
string name;
float balance;
string bname;
string brname;
string ifsc;
public:
Account(string acc, string n)
{
accno = acc;
name = n;
balance = 500;
bname = "SBI";
brname = "BVRM MAIN";
ifsc = "SBI0000818";
}
void withdraw(float amount)
{
balance = balance - amount;
cout<<"Amount withdrawn = "<<amount<<endl;
cout<<"Remaining balance = "<<balance<<endl;
}
void deposit(float amount)
{
balance = balance + amount;
cout<<"Amount deposited = "<<amount<<endl;
cout<<"Remaining balance = "<<balance<<endl;
}
float get_balance()
{
return balance;
}
};
int main()
{
string acc, n;
cout<<"Enter account no: ";
cin>>acc;
cout<<"Enter your name: ";
cin>>n;
Account a1(acc, n);
a1.deposit(5000);
a1.withdraw(2200);
cout<<"Available balance is: "<<a1.get_balance();
return 0;
}
Input and output for the above program are as follows:
Enter account no: 23458
Enter your name: teja
Amount deposited = 5000
Remaining balance = 5500
Amount withdrawn = 2200
Remaining balance = 3300
Available balance is: 3300
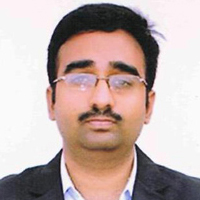
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply