In this article we will learn to implement a Cpp program to create objects of distance class and add them. A C++ program is provided below that adds objects of distance class.
We create a distance class with the following:
- feet and inches as data members
- member function to input distance
- member function to output distance
- member function to add two distance objects
We create two objects of distance class and add them. Program is as follows:
#include <iostream>
using namespace std;
class Distance
{
private:
int feet;
int inches;
public:
void set_distance()
{
cout<<"Enter feet: ";
cin>>feet;
cout<<"Enter inches: ";
cin>>inches;
}
void get_distance()
{
cout<<"Distance is feet= "<<feet<<", inches= "<<inches<<endl;
}
void add(Distance d1, Distance d2)
{
feet = d1.feet + d2.feet;
inches = d1.inches + d2.inches;
feet = feet + (inches / 12);
inches = inches % 12;
}
};
int main()
{
Distance d1, d2, d3;
d1.set_distance();
d2.set_distance();
d3.add(d1, d2);
d3.get_distance();
return 0;
}
Input and output for the above program are as follows:
Enter feet: 3
Enter inches: 8
Enter feet: 4
Enter inches: 9
Distance is feet= 8, inches= 5
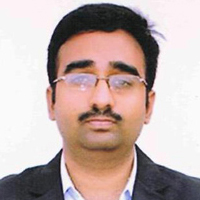
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
wouldn’t it be inches = inches % 10;
Hello. And Bye.
Please using function type demo
What do you mean?