In this article we will learn to implement a C program to reverse an array in place using pointers. A C program is provided below to reverse the given array in place using pointers.
Program is as follows:
#include<stdio.h>
void reversearray(int *p, int n)
{
int *first = p;
int *last = p+n-1;
while(first<last)
{
int temp = *first;
*first = *last;
*last = temp;
first++;
last--;
}
printf("Reversed array elements are: ");
for(int i=0; i<n; i++)
printf("%d ", *p++);
}
int main()
{
int n;
printf("Enter n: ");
scanf("%d", &n);
int a[n];
printf("Enter %d numbers: ");
for(int i=0; i<n; i++)
scanf("%d", &a[i]);
reversearray(a, n);
return 0;
}
Input and output for the above program is as follows:
Enter n: 5
Enter 5 numbers: 6 7 1 3 8
Reversed array elements are: 8 3 1 7 6
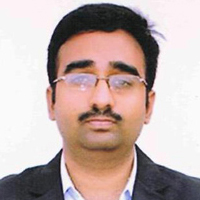
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply