In this article we will learn to implement a C program to print a pattern. The following C program prints the below pattern for n = 4.
1*2*3*4*17*18*19*20
5*6*7*14*15*16
8*9*12*13
10*11
The program is as follows:
#include <stdio.h>
#include <conio.h>
int main()
{
int n = 4;
int lstart = 1;
int rstart = 20 - n + 1;
int dup;
for(int i = n; i >= 1; i--)
{
dup = rstart;
//Prints left half of the pattern
for(int j = 1; j <= i; j++)
{
printf("%d*", lstart);
lstart++;
}
//Prints right half of the pattern
for(int j = 1; j <= i; j++)
{
printf("%d", rstart);
if(j != i)
printf("*");
rstart++;
}
rstart = dup - i + 1;
printf("\n");
}
getch();
return 0;
}
Output of the above program is as follows:
1*2*3*4*17*18*19*20
5*6*7*14*15*16
8*9*12*13
10*11
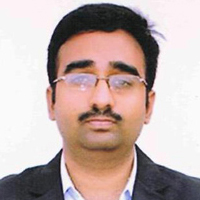
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply