In this article we will learn to implement a C program to generate Fibonacci series using recursion. A C program is provided below to generate a Fibonacci series using both recursive and non-recursive functions.
Program is as follows:
/*
* C program to generate fibonacci sequence using recursive and non-recursive functions
* Author: P.S.SuryaTeja
*/
#include <stdio.h>
#include <conio.h>
#include <math.h>
#include <stdlib.h>
void fib(int n)
{
int a = 0, b = 1, c, count = 3;
if(n == 1)
printf("0");
else if(n == 2)
printf("0 1");
else
{
printf("0 1 ");
while(count <= n)
{
c = a + b;
printf("%d ", c);
a = b;
b = c;
count++;
}
}
}
int rfib(int n)
{
if(n == 1)
return 0;
else if(n == 2)
return 1;
else
{
return rfib(n - 1) + rfib(n - 2);
}
}
int main(int argc, char **argv)
{
int n, count = 3;
printf("Enter a number: ");
scanf("%d", &n);
printf("\nNon-recursive fibonacci sequence upto %d terms: \n", n);
fib(n);
printf("\nRecursive fibonacci sequence upto %d terms: \n", n);
if(n == 1)
printf("0");
else if(n == 2)
printf("0 1");
else
{
printf("0 1 ");
while(count <= n)
{
printf("%d ", rfib(count));
count++;
}
}
getch();
return 0;
}
Input and output for the above program is as follows:
Enter a number: 10
Non-recursive fibonacci sequence upto 10 terms:
0 1 1 2 3 5 8 13 21 34
Recursive fibonacci sequence upto 10 terms:
0 1 1 2 3 5 8 13 21 34
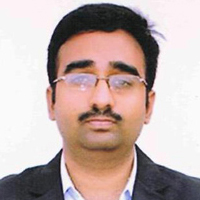
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply