In this article we will learn to implement a C program to concatenate two strings without using library functions. A C program is provided below to join or concatenate two strings without using predefined function like strcat.
Program is as follows:
#include<stdio.h>
#include<string.h>
int main()
{
char str[20];
printf("Enter a string: ");
scanf("%s", str);
char newstr[20];
printf("Enter another string: ");
scanf("%s", newstr);
int newlength = strlen(str) + strlen(newstr);
int i = strlen(str), j = 0;
while(i < newlength)
{
str[i] = newstr[j];
i++;
j++;
}
str[i] = '\0';
printf("Concatenated string is: %s", str);
return 0;
}
Input and output for the above program are as follows:
Enter a string: hello
Enter another string: world
Concatenated string is: helloworld
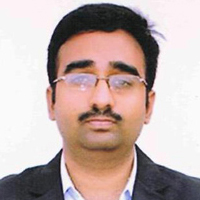
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply