In this article we will learn to implement a C program to compare two arrays using pointers. A C program is provided below for reading two arrays and comparing them using pointers.
Program is as follows:
/*
* C program to compare two arrays using pointers
* Author: P.S.SuryaTeja
*/
#include <stdio.h>
#include <conio.h>
#include <math.h>
#include <stdlib.h>
void compare(int *a1, int *a2, int n)
{
int i, flag = 0;
for(i = 0; i < n; i++)
{
if(*a1 != *a2)
{
flag = 1;
break;
}
a1++;
a2++;
}
if(flag == 1)
printf("\nBoth arrays are not equal");
else
printf("\nBoth arrays are equal");
}
int main(int argc, char **argv)
{
int a1[10], a2[10];
int n, i;
printf("Enter a number between 1 and 10: ");
scanf("%d", &n);
printf("Enter %d numbers for array 1: ", n);
for(i = 0; i < n; i++)
scanf("%d", &a1[i]);
printf("Enter %d numbers for array 2: ", n);
for(i = 0; i < n; i++)
scanf("%d", &a2[i]);
compare(a1, a2, n);
getch();
return 0;
}
Input and output for the above program is as follows:
Enter a number between 1 and 10: 5
Enter 5 numbers for array 1: 1 4 5 2 3
Enter 5 numbers for array 2: 2 3 1 4 5
Both arrays are not equal
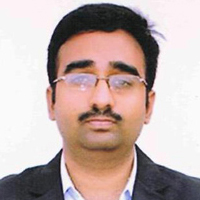
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply