In this article we will learn to implement a C program to compare, concatenate and append strings. A C program is provided below to read two strings and perform comparison, concatenation and append operations.
Program is as follows:
/*
* C program to implement the following string functions using user defined functions:
* i. To concatenate two strings
* ii. To append a string to another string
* iii. To compare two strings
* Author: P.S.SuryaTeja
*/
#include <stdio.h>
#include <conio.h>
#include <math.h>
#include <stdlib.h>
#include <string.h>
void concat(char *str1, char *str2)
{
char str[40];
int str1length, str2length;
int i;
str1length = strlen(str1);
str2length = strlen(str2);
for(i = 0; i < str1length; i++)
{
str[i] = *str1;
str1++;
}
while(i < str1length + str2length)
{
str[i] = *str2;
str2++;
i++;
}
str[i] = '\0';
printf("\nResult of concatenation is: ");
puts(str);
}
void append(char *str1, char *str2)
{
int str1length, str2length;
int i;
char *tempstr;
tempstr = str1;
str1length = strlen(str1);
str2length = strlen(str2);
for(i = 0; i < str1length; i++)
str1++;
for(i = 0; i < str2length; i++)
{
*str1 = *str2;
str1++;
str2++;
}
*str1 = '\0';
printf("\nResult of appending is: ");
puts(tempstr);
}
void compare(char *str1, char *str2)
{
int str1length, str2length;
int i, flag = 0;
str1length = strlen(str1);
str2length = strlen(str2);
if(str1length != str2length)
printf("\nBoth strings are not equal");
else
{
for(i = 0; i < str1length; i++)
{
if(*str1 != *str2)
flag = 1;
str1++;
str2++;
}
if(flag == 0)
printf("\nBoth strings are equal");
else
{
printf("\nBoth strings are not equal");
}
}
}
int main(int argc, char **argv)
{
char str1[20], str2[20], str3[20];
printf("Enter string 1: ");
gets(str1);
printf("Enter string 2: ");
gets(str2);
strcpy(str3,str1);
printf("\n");
concat(str1, str2);
printf("\n");
append(str1, str2);
printf("\n");
compare(str3, str2);
getch();
return 0;
}
Input and output for the above program is as follows:
Enter string 1: hai
Enter string 2: hai
Result of concatenation is: haihai
Result of appending is: haihai
Both strings are equal
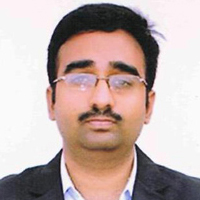
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply