In this article we will learn to implement a C program to add and multiply two compatible matrices. A C program is provided below to read two matrices, add them and then multiply them.
Program is as follows:
//C program to add and multiply two compatible matrices
#include<stdio.h>
#include<conio.h>
void main()
{
int a[3][3], b[3][3], c[3][3]={0}, d[3][3]={0};
int i,j,k,m,n,p,q;
printf("Enter no. of rows and columns in matrix A: ");
scanf("%d%d",&m,&n);
printf("Enter no. of rows and columns in matrix B: ");
scanf("%d%d",&p,&q);
if(m!=p || n!=q)
{
printf("Matrix Addition is not possible");
return;
}
else if(n!=p)
{
printf("Matrix Multiplication is not possible");
return;
}
else
{
printf("Enter elements of matrix A: ");
for(i=0;i<m;i++)
for(j=0;j<n;j++)
scanf("%d", &a[i][j]);
printf("Enter elements of matrix B: ");
for(i=0;i<p;i++)
for(j=0;j<q;j++)
scanf("%d", &b[i][j]);
//Matrix Addition
for(i=0;i<m;i++)
for(j=0;j<n;j++)
c[i][j] = a[i][j] + b[i][j];
printf("\nResult of Matirx Addition:\n");
for(i=0;i<m;i++)
{
for(j=0;j<n;j++)
printf("%d ", c[i][j]);
printf("\n");
}
//Matrix Multiplication
for(i=0;i<m;i++)
for(j=0;j<q;j++)
for(k=0;k<p;k++)
d[i][j] += a[i][k]*b[k][j];
printf("\nResult of Matirx Multiplication:\n");
for(i=0;i<m;i++)
{
for(j=0;j<q;j++)
printf("%d ", d[i][j]);
printf("\n");
}
}
getch();
}
Input and output for the above program is as follows:
Enter no. of rows and columns in matrix A: 3 3
Enter no. of rows and columns in matrix B: 3 3
Enter elements of matrix A:
1 1 1
2 2 2
3 3 3
Enter elements of matrix B:
2 2 2
2 2 2
2 2 2
Result of Matirx Addition:
3 3 3
4 4 4
5 5 5
Result of Matirx Multiplication:
6 6 6
12 12 12
18 18 18
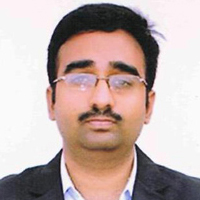
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
Leave a Reply