In this article we will learn to implement a Java program that checks whether a given string is a palindrome or not. A Java program is provided below that reads a string, checks whether it is a palindrome or not.
Program is as follows:
import java.util.*;
class Palindrome
{
void isPalindrome(String str)
{
String org, rev;
StringBuffer buff1, buff2;
buff1 = new StringBuffer(str);
org = new String(buff1);
buff2 = buff1.reverse();
rev = new String(buff2);
if(org.equals(rev))
{
System.out.println("Entered string is a palindrome");
}
else
{
System.out.println("Entered string is not a palindrome");
}
}
}
class Driver
{
public static void main(String[] args)
{
Scanner s = new Scanner(System.in);
System.out.print("Enter a string: ");
String str = s.next();
Palindrome p = new Palindrome();
p.isPalindrome(str);
}
}
Input and output for the above program is as follows:
Enter a string: madam
Entered string is a palindrome
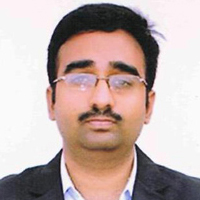
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
thank you