In this article we will learn to implement a C program to read and print a matrix using pointers. A C program is provided below to read and print a matrix using pointers and dynamic memory allocation.
Program is as follows:
#include<stdio.h>
#include<stdlib.h>
int main()
{
int m, n;
printf("Enter no. of rows and columns: ");
scanf("%d%d", &m, &n);
int **a;
//Allocate memory to matrix
a = (int **) malloc(m * sizeof(int *));
for(int i=0; i<m; i++)
{
a[i] = (int *) malloc(n * sizeof(int));
}
//Read elements into matrix
printf("Enter matrix elements: ");
for(int i=0; i<m; i++)
{
for(int j=0; j<n; j++)
{
scanf("%d", &a[i][j]);
}
}
//Print elements in the matrix
printf("Matrix elements are: \n");
for(int i=0; i<m; i++)
{
for(int j=0; j<n; j++)
{
printf("%d ", a[i][j]);
}
printf("\n");
}
//Dellocating memory of matrix
for(int i=0; i<m; i++)
{
free(a[i]);
}
free(a);
return 0;
}
Input and output for the above program is as follows:
Enter no. of rows and columns: 3 3
Enter matrix elements:
1 2 3
4 5 6
7 8 9
Matrix elements are:
1 2 3
4 5 6
7 8 9
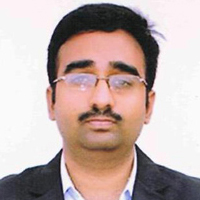
Suryateja Pericherla, at present is a Research Scholar (full-time Ph.D.) in the Dept. of Computer Science & Systems Engineering at Andhra University, Visakhapatnam. Previously worked as an Associate Professor in the Dept. of CSE at Vishnu Institute of Technology, India.
He has 11+ years of teaching experience and is an individual researcher whose research interests are Cloud Computing, Internet of Things, Computer Security, Network Security and Blockchain.
He is a member of professional societies like IEEE, ACM, CSI and ISCA. He published several research papers which are indexed by SCIE, WoS, Scopus, Springer and others.
why?